How to Automate Your YouTube Content Creation with AI: Building an End-to-End AI Video Generator

In today's digital landscape, creating engaging YouTube content consistently can be challenging and time-consuming. What if you could automate this process, generating professional-quality videos from just a topic idea? That's exactly what we've built with our AI Video Generator, a tool that lets you create complete YouTube videos with minimal human intervention.
In this comprehensive guide, I'll walk you through how we built this system, combining multiple AI technologies including OpenAI's GPT, Stability AI for image and video generation, and Murf for realistic text-to-speech. By the end, you'll understand how each component works together to transform a simple topic into a polished video ready for YouTube.
See It In Action: Video Demo
Before diving into the technical details, check out this demo where I use the AI Video Generator to create a complete video on "Global Warming":
Watch the AI Video Generator Demo
In this demo, you'll see:
- How quickly the system generates a complete script from just the topic "Global Warming"
- The natural-sounding voice synthesis switching between male and female narrators
- The AI-generated visuals that match the script content
- The final assembled video with synchronized audio and animated visuals
This is all created automatically with minimal human intervention—just enter a topic and the system handles the rest!
Prerequisites:
Before diving into building your own AI-powered YouTube video generator, ensure you have the following tools, accounts, and knowledge in place:
- Python and Node.js installed
- The backend is built using Python (Flask), and the frontend uses React with Vite. Make sure you have Python 3.8+ and Node.js 16+ installed on your system.
- API Keys
- OpenAI API Key: Required for script generation using GPT. Sign up at OpenAI.
- Stability AI API Key: Needed for image and video generation. Get it from Stability AI.
- Murf AI API Key: Used for text-to-speech conversion. Sign up at Murf AI.
- Basic knowledge of Python and JavaScript
- Familiarity with Python (for backend development) and JavaScript/React (for frontend development) will help you understand and customize the code.
- FFmpeg (for Audio/Video Processing)
- MoviePy (used for video assembly) requires FFmpeg. Install it from FFmpeg's official site or you can install it using:
- Windows: choco install ffmpeg
- MacOS: brew install ffmpeg
- Linux: sudo apt-get install ffmpeg
System Overview
Our AI Video Generator follows this workflow:
- User Input: Enter a simple topic (e.g., "Global Warming")
- Script Generation: GPT generates a script with voice parts and image prompts
- Audio Creation: Murf AI converts the script into natural-sounding voice tracks
- Visual Generation: Stability AI creates images and animates them
- Video Assembly: The system combines everything into the final video
Understanding the Project Structure:
{{qq-border-start}}
youtube-video-automation/
│
├── backend/
│ ├── app.py
│ └── requirements.txt
│
├── frontend/
│ ├── src/
│ │ ├── assets/
│ │ │ └── styles/
│ │ │ └── App.css
│ │ ├── components/
│ │ │ └── VideoGenerator.jsx
│ │ ├── services/
│ │ │ └── api.js
│ │ ├── App.jsx
│ │ ├── main.jsx
│ │ └── index.html
│
├── venv/
└── .gitignore
{{qq-border-end}}
Let's dive into how each part works!
Backend Development
The backend serves as the engine of our application, handling the heavy lifting of processing data, communicating with external APIs, and generating the final video. Let's examine each major component:
Setting Up the Flask Server
{{qq-border-start}}
from flask import Flask, jsonify, request, send_file, send_from_directory
from flask_cors import CORS
import os
app = Flask(__name__, static_folder='static')
CORS(app, resources={r"/api/*": {"origins": "http://localhost:5173"}})
# Temporary directory to store processing files
TEMP_DIR = "temp_files"
os.makedirs(TEMP_DIR, exist_ok=True)
{{qq-border-end}}
Our backend application uses Flask, which provides a lightweight framework ideal for creating APIs. The code above sets up several key components:
First, we initialize a Flask application and configure Cross-Origin Resource Sharing (CORS). This is crucial because our frontend and backend run on different ports (5173 for the React frontend and the default 5000 for Flask). Without proper CORS settings, the browser would block requests between these components due to security restrictions.
We also create a temporary directory (temp_files) to store all the intermediate and final files generated during video creation - including images, audio clips, and video segments. Making this directory with exist_ok=True ensures our app won't crash if the directory already exists from a previous run.
Managing Text-to-Speech with Murf AI
{{qq-border-start}}
@app.route('/api/murf-token', methods=['GET'])
def get_murf_token():
try:
murf = Murf(api_key=os.getenv('MURF_API_KEY'))
token = murf.auth.generate_token()
return jsonify({
'token': token.token,
'expiry': token.expiry_in_epoch_millis
})
except Exception as e:
return jsonify({'error': str(e)}), 500
{{qq-border-end}}
This endpoint demonstrates an important security practice: never exposing your API keys directly to the frontend. Instead of sending the API key to the browser (where it could potentially be compromised), our backend serves as a secure intermediary. When the frontend needs to interact with Murf, it first requests a temporary token from our backend.
The backend, which safely stores the API key in environment variables, generates this token and sends it back. This token is short-lived and has limited permissions, making it much safer to use in a browser environment. The response includes not only the token itself but also its expiry time, allowing the frontend to know when it needs to request a fresh token.
And then comes our “generate-speech” endpoint:
{{qq-border-start}}
@app.route('/api/generate-speech', methods=['POST'])
async def generate_speech():
data = request.json
token = data.get('token')
script = data.get('script')
if not token or not script:
logger.error('Token and script are required')
return jsonify({'error': 'Token and script are required'}), 400
async def generate_audio_for_line(line):
logger.debug(f"Generating audio for line: {line}") # Log the line being processed
async with aiohttp.ClientSession() as session:
async with session.post('https://api.murf.ai/v1/speech/generate',
headers={'Content-Type': 'application/json', 'token': token},
json=line) as response:
if response.status != 200:
error_text = await response.text()
logger.error(f"Error generating speech: {error_text}") # Log the error
return None
audio_response = await response.json()
logger.info(f"Successfully generated audio for line: {line}") # Log success
return audio_response['audioFile']
tasks = [generate_audio_for_line(line) for line in script]
audio_segments = await asyncio.gather(*tasks)
# Filter out None values
audio_segments = [segment for segment in audio_segments if segment is not None]
return jsonify(audio_segments)
{{qq-border-end}}
This endpoint handles the conversion of our script into natural-sounding speech using the Murf API. Let's break down how it works:
First, we extract the token and script from the incoming JSON request. The script is an array of objects, each containing the voice ID and text to be converted to speech. We perform basic validation to ensure both the token and script are provided.
The core of this endpoint is the generate_audio_for_line asynchronous function, which handles the conversion of a single line of script to audio. We're using Python's aiohttp library, which enables non-blocking HTTP requests - perfect for API calls that might take some time to complete.
For each line in the script, we create an asynchronous task that:
- Opens a session with the Murf API
- Sends the line along with the authentication token
- Waits for and processes the response
- Returns the URL of the generated audio file, or None if there was an error
The magic happens with asyncio.gather(*tasks), which executes all these tasks concurrently and waits for all of them to complete. This is much faster than processing each line sequentially, especially for scripts with multiple lines.
Finally, we filter out any failed audio generation attempts (represented by None values) and return an array of audio file URLs as a JSON response. This robust approach ensures that even if one line fails to process, the rest of the generation can continue.
Generating Images with Stability AI
{{qq-border-start}}
@app.route('/api/generate-images', methods=['POST'])
def generate_images():
try:
data = request.json
prompt = data.get('prompt', '')
if not prompt:
return jsonify({'error': 'No prompt provided'}), 400
# Call Stability.ai image generation API
response = requests.post(
'https://api.stability.ai/v2beta/stable-image/generate/core',
headers={
'Authorization': f'Bearer {STABILITY_API_KEY}',
'Accept': 'image/*'
},
data={
'prompt': prompt,
'output_format': 'png'
}
)
if response.status_code != 200:
return jsonify({
'error': f'Stability API error: {response.text}',
'status_code': response.status_code
}), 500
image_data = response.content
# Generate a unique filename using UUID
unique_filename = f"generated_image_{uuid.uuid4()}.png"
image_file_path = os.path.join(TEMP_DIR, unique_filename)
with open(image_file_path, 'wb') as image_file:
image_file.write(image_data)
# Resize the image to supported dimensions
resized_image_path = os.path.join(TEMP_DIR, f'resized_{unique_filename}')
with Image.open(image_file_path) as img:
img = img.resize((1024, 576)) # Resize to 16:9 aspect ratio
img.save(resized_image_path)
return jsonify({'image_path': resized_image_path})
except Exception as e:
return jsonify({
'error': str(e),
'message': 'Failed to generate images'
}), 500
{{qq-border-end}}
This endpoint handles image generation, a critical component of our video creation process. The function takes a text prompt from the frontend and uses Stability AI's API to generate a matching visual.
The process starts with basic input validation - ensuring we actually have a prompt to work with. Then, we format a request to Stability AI's image generation API, which specializes in creating high-quality, detailed images from text descriptions.
After receiving the image, we handle several important details that make the difference between amateur and professional-quality videos:
- Unique Filenames: Using UUID (Universally Unique Identifier) ensures that even if multiple users are generating videos simultaneously, their files won't conflict with each other.
- Image Resizing: We resize all images to a 1024×576 resolution, which maintains a 16:9 aspect ratio - the standard for YouTube videos. This ensures our final video looks professional without letterboxing or stretching.
By handling these details on the backend, we simplify the frontend experience while ensuring consistent, high-quality output.
Creating the Video: The Heart of the Process
This endpoint is the heart of our application, where all the components come together to create the final video. It's a multi-step process that transforms static images and audio clips into a cohesive, professional video.
Step 1: Converting Static Images to Videos
{{qq-border-start}}
@app.route('/api/create-video', methods=['POST'])
def create_video():
try:
data = request.json
image_paths = data.get('image_paths') # Expect a list of image paths
audio_data_list = data.get('audioData') # Expect a list of audio blobs
if not image_paths or len(image_paths) == 0:
return jsonify({'error': 'Missing image paths'}), 400
if not audio_data_list or len(audio_data_list) == 0:
return jsonify({'error': 'Missing audio data'}), 400
# Step 1: Generate videos from each image
video_paths = []
for image_path in image_paths:
video_response = requests.post(
"https://api.stability.ai/v2beta/image-to-video",
headers={
"authorization": f"Bearer {STABILITY_API_KEY}"
},
files={
"image": open(image_path, "rb")
},
data={
"seed": 0,
"cfg_scale": 1.8,
"motion_bucket_id": 127
},
)
if video_response.status_code != 200:
raise Exception(f"Error generating video: {video_response.json()}")
generation_id = video_response.json().get('id')
# Check the status of the video generation
while True:
time.sleep(10) # Wait for 10 seconds before checking again
status_response = requests.get(
f"https://api.stability.ai/v2beta/image-to-video/result/{generation_id}",
headers={
'accept': "video/*",
'authorization': f"Bearer {STABILITY_API_KEY}"
},
)
if status_response.status_code == 202:
# Still processing
pass
elif status_response.status_code == 200:
video_data = status_response.content
if not video_data:
raise Exception("Received empty video data.")
# Save the generated video
video_path = os.path.join(TEMP_DIR, f'video_{generation_id}.mp4')
with open(video_path, 'wb') as file:
file.write(video_data)
video_paths.append(video_path)
break
else:
raise Exception(f"Error checking video generation status: {status_response.json()}"
{{qq-border-end}}
We start by transforming each static image into a short video clip using Stability AI's image-to-video API. This creates subtle movements in each scene that make the video more engaging than a simple slideshow.
The API works asynchronously - we submit our request and then poll periodically to check if the processing is complete. This polling approach (checking every 10 seconds) is a common pattern for handling long-running operations in web applications. We continue checking until the status code changes from 202 (processing) to 200 (complete), then save the video segment.
Step 2: Audio Processing and Combination
{{qq-border-start}}
# Step 2: Combine audio files
combined_audio = AudioSegment.empty()
for audio_data in audio_data_list:
if isinstance(audio_data, str):
header, base64_data = audio_data.split(',', 1)
audio_data = base64.b64decode(base64_data)
temp_audio_path = os.path.join(TEMP_DIR, 'temp_audio.mp3')
with open(temp_audio_path, 'wb') as audio_file:
audio_file.write(audio_data)
audio_segment = AudioSegment.from_file(temp_audio_path)
combined_audio += audio_segment
combined_audio_path = os.path.join(TEMP_DIR, 'combined_audio.mp3')
combined_audio.export(combined_audio_path, format='mp3')
{{qq-border-end}}
Here, we process the audio data received from the frontend. The audio comes in as base64-encoded strings, which we decode and write to temporary files. Using the pydub library's AudioSegment class, we load each audio file and combine them sequentially into a single continuous audio track.
Step 3: Final Video Assembly
{{qq-border-start}}
# Step 3: Merge combined audio and all videos
final_output_path = os.path.join(TEMP_DIR, 'final_output.mp4')
video_clips = [VideoFileClip(video_path) for video_path in video_paths]
final_video = concatenate_videoclips(video_clips)
audio_clip = AudioFileClip(combined_audio_path)
final_video = final_video.set_audio(audio_clip)
# Loop the last video if audio is longer
if audio_clip.duration > final_video.duration:
final_video = final_video.loop(duration=audio_clip.duration)
# Write the final output video
final_video.write_videofile(final_output_path, codec='libx264', audio_codec='aac')
return jsonify({'status': 'success', 'output_path': final_output_path})
except Exception as e:
return jsonify({
'error': str(e),
'message': 'Failed to create video'
}), 500
{{qq-border-end}}
This final step uses MoviePy, a powerful Python library for video editing. We load all our video clips, concatenate them in sequence, and attach the combined audio track to create the final video.
A particularly clever feature is how we handle timing mismatches: if the audio is longer than the combined video (which often happens), we loop the last video segment to match the audio duration. This ensures the video doesn't end while the narration is still playing.
Finally, we encode the video using industry-standard codecs (H.264 for video and AAC for audio) to ensure compatibility with platforms like YouTube and social media.
Serving the Final Video
{{qq-border-start}}
@app.route('/videos/<path:filename>', methods=['GET'])
def serve_video(filename):
return send_from_directory(TEMP_DIR, filename)
{{qq-border-end}}
This simple but crucial endpoint provides access to the generated videos. It uses Flask's send_from_directory function to serve files from our temporary directory.
By exposing this endpoint, our frontend can directly access the final video for playback and download. The <path:filename> part in the route is a URL parameter that captures the filename requested by the frontend, allowing for dynamic access to any file in our temporary directory.
Frontend Development
Now let's explore the frontend components that provide the user interface for our AI Video Generator. The frontend is built with React, making it interactive and responsive.
The Main Video Generator Component
This React component manages the entire video generation process from the user's perspective. Think of it as the control center that coordinates all the different AI services and keeps the user informed about what's happening.
{{qq-border-start}}
import React, { useState } from 'react';
import { generateScripts, generateMurfAudio, generateStabilityImages, createVideo } from '../services/api';
import '../assets/styles/App.css';
const VideoGenerator = () => {
const [topic, setTopic] = useState('');
const [status, setStatus] = useState('');
const [progress, setProgress] = useState(0);
const [videoUrl, setVideoUrl] = useState(null);
const [error, setError] = useState(null);
const [isGenerating, setIsGenerating] = useState(false);
{{qq-border-end}}
We use React's useState hooks to manage several pieces of state:
- topic: The user's input topic for the video
- status: A message describing the current stage of generation
- progress: A number (0-100) representing completion percentage
- videoUrl: The URL of the final video once it's ready
- error: Any error messages that occur during generation
- isGenerating: A boolean flag indicating whether generation is in progress
The heart of this component is the handleGenerate function,
{{qq-border-start}}
const handleGenerate = async () => {
if (!topic.trim()) {
setError('Please enter a topic');
return;
}
try {
setIsGenerating(true);
setError(null);
setVideoUrl(null);
setProgress(10);
// Step 1: Create script
setStatus('Creating script with AI...');
const { murf, stability } = await generateScripts(topic);
setProgress(25);
// Step 2: Generate audio
setStatus('Generating voice tracks...');
const audioFiles = await generateMurfAudio(murf);
setProgress(50);
// Step 3: Generate images
setStatus('Creating visuals... (may take 1-2 minutes)');
const imageData = await generateStabilityImages(stability);
setProgress(75);
// Step 4: Create video from images and audio
setStatus('Cooking your video, take a tea break till then...(may take 5-6 minutes)');
const finalVideoPath = await createVideo(imageData, audioFiles);
// Set the video source to the URL served by the backend
const videoUrl = `http://127.0.0.1:5000/videos/final_output.mp4`;
setVideoUrl(videoUrl);
setStatus('');
setProgress(100);
} catch (error) {
console.error('Video generation error:', error);
setError(`Error: ${error.message || 'Something went wrong'}`);
setStatus('Generation failed');
} finally {
setIsGenerating(false);
}
};
const resetGenerator = () => {
setVideoUrl(null);
setStatus('');
setProgress(0);
setError(null);
};
// Component JSX...
};
{{qq-border-end}}
This function orchestrates the four-step process of video creation:
- Script Generation: We call our API service to get GPT to create a script with voice lines and image prompts
- Audio Generation: We convert the script to voice audio using Murf
- Image Generation: We create visuals based on the prompts using Stability AI
- Video Assembly: We send everything to the backend to be combined into the final video
Throughout this process, we update the status message and progress percentage to keep the user informed. This is crucial because video generation can take several minutes, and without feedback, users might think the application has frozen.
We also have error handling throughout the process. If anything goes wrong at any stage, we catch the error, display a user-friendly message, and reset the generation state. This ensures users aren't left hanging if something unexpected happens.
The resetGenerator function provides a way for users to start over after generating a video or encountering an error. This improves the user experience by making the application feel more responsive and less like a one-shot process.
API Service: Communicating with the Backend
{{qq-border-start}}
import axios from 'axios';
const GPT_PROMPT = `Generate JSON with:
1. 'murf' key: Array of vocal objects (voiceId, text)
- Use 'en-US-amara' (female) and 'en-US-miles' (male)
- Keep dialogues natural and engaging
- This full audio should be enough for a 30 - 40 sec video (not less, not more)
2. 'stability' key: Array of 7 image prompts
- Each prompt should be under 12 words
- Focus on visual elements and composition
- Each prompt should be a complete one in itself.
Example of expected response:
{{
"murf": [
{{
"voiceId": "en-US-amara",
"text": "Welcome to our video on the wonders of nature."
}},
{{
"voiceId": "en-US-miles",
"text": "In this video, we will explore the beauty of the world around us."
}}
],
"stability": ["A serene landscape with mountains and a lake.", "A scenic beauty of himalayan sunrise", ...]
}}
Topic: {TOPIC}`;
export const generateScripts = async (topic) => {
const response = await fetch('https://api.openai.com/v1/chat/completions', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${import.meta.env.VITE_OPENAI_KEY}`
},
body: JSON.stringify({
model: 'gpt-4o-mini',
response_format: { type: 'json_object' },
messages: [{
role: 'user',
content: GPT_PROMPT.replace('{TOPIC}', topic)
}],
temperature: 0.7
})
});
const data = await response.json();
return JSON.parse(data.choices[0].message.content);
};
{{qq-border-end}}
This function showcases one of the most fascinating aspects of our application: using AI to generate the creative content for our video. Instead of requiring users to manually write scripts and image descriptions, we leverage GPT to automate this creative process.
The key to getting consistent, usable results from GPT lies in our prompt engineering. We've crafted a detailed prompt that specifies:
- The exact format we want: A JSON object with specific keys
- Voice specification: Alternating between male and female voices for variety
- Content guidelines: Natural dialogue, appropriate length for a 30-40 second video
- Image prompt parameters: Brief, visually-focused descriptions
We also include an example of the expected output format, which helps GPT understand exactly what we need. This is a powerful technique in prompt engineering called "few-shot learning" - by showing an example of what we want, we dramatically improve the quality and consistency of the results.
The actual API call is straightforward: we send our constructed prompt to OpenAI's API, requesting the gpt-4o-mini model with a temperature of 0.7 (balancing creativity and consistency) and explicitly requesting JSON output format.
The result is a complete script and set of image prompts tailored to the user's topic, ready to be passed to the next stages of our pipeline.
Audio Generation with Murf AI
{{qq-border-start}}
export const generateMurfAudio = async (script) => {
const tokenRes = await fetch('http://127.0.0.1:5000/api/murf-token');
if (!tokenRes.ok) {
throw new Error('Failed to fetch token');
}
const { token } = await tokenRes.json();
const response = await fetch('http://127.0.0.1:5000/api/generate-speech', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ token, script }) // Send token and entire script to backend
});
if (!response.ok) {
const errorText = await response.text();
console.error('Error generating audio:', errorText);
throw new Error('Failed to generate audio');
}
const audioSegments = await response.json(); // Get the final array of audio segments
return audioSegments; // Return the array of audio segments
};
{{qq-border-end}}
This function handles the conversion of our script into natural-sounding speech using Murf's text-to-speech API. It demonstrates our secure token-based architecture for working with external APIs.
First, we request a secure token from our backend using the /api/murf-token endpoint. Once we have this token, we can proceed with the speech generation process.
Instead of making individual requests for each line of the script, we send the entire script array along with the token to our backend's /api/generate-speech endpoint. This approach has several advantages:
- It reduces the number of HTTP requests between the frontend and backend
- It allows our backend to handle the parallel processing of multiple script lines
- It simplifies error handling and debugging
The backend processes all script lines and returns an array of URLs pointing to the generated audio files. This array of audio segments is exactly what we need for the next step - combining the audio with visuals to create our final video.
This approach showcases modern web development practices: offloading complex or resource-intensive operations to the backend while keeping the frontend focused on user interaction and presentation.
Image Generation and Video Creation
These two functions i.e. generateStabilityImages and createVideo , handle the visual aspects of our video creation process and the final assembly. Like our audio generation function, they demonstrate efficient parallel processing and robust error handling.
1. generateStabilityImages
{{qq-border-start}}
export const generateStabilityImages = async (prompts) => {
const imagePromises = prompts.map(async (prompt) => {
const response = await axios.post('http://127.0.0.1:5000/api/generate-images', { prompt });
if (response.status !== 200) {
throw new Error(`Image generation failed: ${response.statusText}`);
}
return response.data.image_path;
});
return Promise.all(imagePromises);
};
export const createVideo = async (imagePaths, audioBlobs) => {
try {
if (!Array.isArray(audioBlobs)) {
audioBlobs = [audioBlobs];
}
const formData = {
image_paths: imagePaths,
audioData: audioBlobs.map(blob => {
return blob instanceof Blob ? URL.createObjectURL(blob) : blob;
})
};
const response = await axios.post('http://127.0.0.1:5000/api/create-video', formData, {
headers: { 'Content-Type': 'application/json' },
timeout: 1200000 // 20 minutes
});
if (response.status !== 200) {
throw new Error(`Video creation failed: ${response.statusText}`);
}
return response.data.output_path;
} catch (error) {
throw new Error('Video processing error: ' + (error.response?.data?.error || error.message));
}
};
{{qq-border-end}}
The generateStabilityImages function takes an array of prompts and processes them in parallel. For each prompt, it sends a request to our backend API, which in turn communicates with Stability AI to generate an image matching the description. The result is an array of image paths that can be used in the final video.
2. createVideo
{{qq-border-start}}
export const createVideo = async (imagePaths, audioBlobs) => {
try {
if (!Array.isArray(audioBlobs)) {
audioBlobs = [audioBlobs];
}
const formData = {
image_paths: imagePaths,
audioData: audioBlobs.map(blob => {
return blob instanceof Blob ? URL.createObjectURL(blob) : blob;
})
};
const response = await axios.post('http://127.0.0.1:5000/api/create-video', formData, {
headers: { 'Content-Type': 'application/json' },
timeout: 1200000 // 20 minutes
});
if (response.status !== 200) {
throw new Error(`Video creation failed: ${response.statusText}`);
}
return response.data.output_path;
} catch (error) {
throw new Error('Video processing error: ' + (error.response?.data?.error || error.message));
}
};
{{qq-border-end}}
The createVideo function is the culmination of our entire process, sending all the prepared assets (image paths and audio data) to the backend for assembly. This function includes several important features:
- Data Normalization: It ensures that audio data is always treated as an array, even if only a single audio segment is provided.
- Format Conversion: It handles both Blob objects and base64 strings, converting Blobs to URLs when needed.
- Extended Timeout: Since video processing can take several minutes, we set a generous 20-minute timeout (1,200,000 ms) to ensure the request doesn't time out prematurely.
- Error Handling: We catch any errors that occur during the process and transform them into user-friendly error messages that can be displayed in the UI.
Together, these functions complete our end-to-end pipeline, turning the user's topic into a fully-realized video through a series of API calls and data transformations.
Putting It All Together
Imagine a factory where raw ideas go in one end and polished videos come out the other. That's exactly what our AI Video Generator achieves a seamless assembly line of creativity powered by cutting-edge AI. Here's how the magic happens:
- Script Generation: GPT-4o-mini acts as your digital screenwriter, crafting compelling narratives from a single topic. Whether it's a tech explainer or a travel vlog, GPT structures the story with engaging hooks, clear explanations, and natural dialogue.
- Voice Synthesis: Murf's API steps in as your voice actor, transforming text into lifelike speech. Our backend efficiently orchestrates this process, converting multiple script lines into audio simultaneously for optimal performance.
- Image Generation: Stability AI brings your script to life visually. From futuristic cityscapes to serene nature shots, it generates high-quality images that match the narrative perfectly.
- Animation: Static images get a cinematic upgrade. Stability AI's image-to-video feature adds subtle motion like drifting clouds or flickering lights making every scene dynamic and engaging.
- Assembly: Finally, the system stitches everything together. Audio, visuals, and animations are synchronized into a cohesive video, complete with smooth transitions and professional pacing.
The result? A fully automated pipeline that turns "Explain quantum computing" into a sleek, shareable video in minutes. It's not just automation it's creativity at scale.
Future Enhancements
While the current system is already a game-changer, the future holds even more exciting possibilities:
- Longer Videos: Expand the framework to support 10-minute deep dives or even hour-long documentaries, complete with chapter markers and advanced storytelling techniques.
- Style Customization: Let users choose visual styles (e.g., cyberpunk, watercolor, or photorealistic) and voice preferences (e.g., energetic, calming, or authoritative) to match their brand or mood.
- Music Integration: Add royalty-free background music or AI-generated soundtracks that adapt to the video’s tone and pacing.
- Templates: Pre-built templates for different content types—product demos, educational explainers, or viral shorts could make video creation even faster and more accessible.
- Direct Publishing: Integrate with platforms like YouTube, TikTok, and Instagram to schedule and publish videos directly from the app.
These enhancements will transform the AI Video Generator from a tool into a full-fledged content creation studio one that’s accessible to anyone with an idea.
Conclusion
Building an AI-powered video generator isn’t just a technical achievement it’s a glimpse into the future of content creation. What once required a team of writers, designers, and editors can now be accomplished with a few lines of code and a well-crafted prompt.
This technology is a great equalizer. Whether you’re a solo creator, a small business, or an educator, you can now produce professional-quality videos without expensive equipment or technical expertise. It’s not about replacing human creativity it’s about amplifying it.
As AI continues to evolve, tools like this will become even more powerful and intuitive. The future of content creation isn’t just automated—it’s intelligent, accessible, and endlessly creative.
Ready to Build Your Own?
The future is here, and it’s yours to shape. Clone the repository, plug in your API keys, and start turning your ideas into videos today. Whether you’re creating content for YouTube, social media, or your next big project, the AI Video Generator is your shortcut to professional-quality results.
Let’s revolutionize content creation—one automated video at a time. 🚀
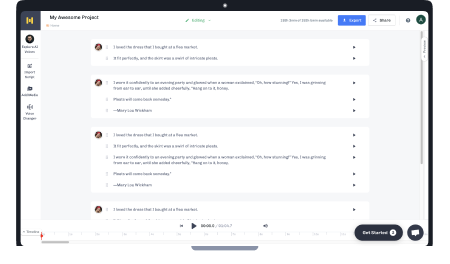